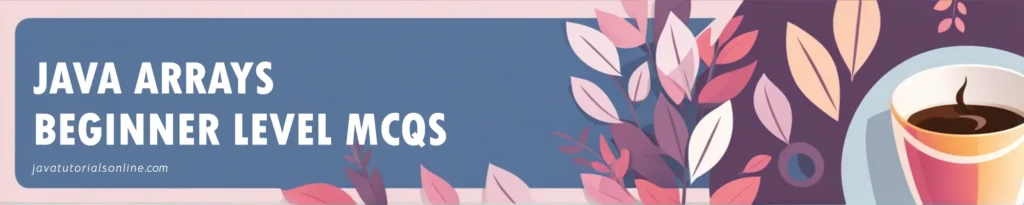
Java Arrays are fundamental for storing multiple values in a single variable. Whether you’re new to Java or looking to strengthen your basics, practicing Java Arrays MCQs can be incredibly beneficial for solidifying your understanding and improving your coding skills.
Here is the basic syntax of an array in Java:
datatype[] arrayName = new datatype[size];
You can also explore more about arrays on Oracle’s official Java documentation.
Java Arrays MCQs (Easy)
Now, let’s test your knowledge with some multiple-choice questions focused on Java arrays.
What is the default value of an uninitialized element in an integer array in Java?
View Answer
Correct Answer: C
In Java, the default value for integer array elements is 0 when the array is initialized. Option 0 is correct.
Which of the following correctly declares a 2D array in Java?
View Answer
Correct Answer: A
In Java, a 2D array can be declared using any of the listed syntax. All of them are valid ways to declare a 2D array.
What is the output of the following code?
int[] arr = {1, 2, 3, 4};
System.out.println(arr[2]);
View Answer
Correct Answer: C
Array indexing starts from 0 in Java. The element at index 2 is 3, so option 2 is correct.
How do you initialize an array with 5 elements in Java?
View Answer
Correct Answer: C
Both `int[] arr = new int[5];` and `int arr[] = new int[5];` are valid ways to initialize an array with 5 elements.
What will be the output of the following code?
int[] arr = {10, 20, 30};
System.out.println(arr[3]);
View Answer
Correct Answer: C
Array indices in Java are 0-based. Trying to access index 3 will throw an ArrayIndexOutOfBoundsException, as the valid indices are 0, 1, and 2.
Which method is used to copy the elements of one array to another in Java?
View Answer
Correct Answer: C
The `Arrays.copyOf()` method is used to copy the elements of an array to another array in Java.
Which of the following statements is true about Java arrays?
View Answer
Correct Answer: B
Arrays in Java are objects. They are passed by reference, not by value, and their size is fixed once initialized.
What is the output of the following code?
int[] arr = {1, 2, 3};
System.out.println(arr.length);
View Answer
Correct Answer: B
The length of the array `arr` is 3 since it contains three elements. Option 2 is correct.
What is the result of the following code?
int[] arr = {10, 20, 30};
arr[1] = 50;
System.out.println(arr[1]);
View Answer
Correct Answer: C
The value at index 1 is updated to 50, so the output will be 50.
Which of the following is true about a jagged array in Java?
View Answer
Correct Answer: D
A jagged array in Java is an array of arrays where each row can have a different number of columns.
What is the output of the following code?
int[] arr = {1, 2, 3};
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
View Answer
Correct Answer: A
The code correctly prints all elements of the array in order: `1 2 3`.
Which of the following will result in an ArrayIndexOutOfBoundsException?
View Answer
Correct Answer: C
Array indices in Java are 0-based. Therefore, attempting to access index 5 in an array of size 5 will throw an ArrayIndexOutOfBoundsException.
How would you initialize an array of size 3 to store the values 1, 2, and 3?
View Answer
Correct Answer: B
The correct syntax to initialize an array with values is `int[] arr = {1, 2, 3};`.
What is the output of the following code?
int[] arr = {5, 10, 15};
for (int i = 0; i < arr.length; i++) {
if (i == 1) continue;
System.out.print(arr[i] + " ");
}
View Answer
Correct Answer: C
The `continue` statement skips the iteration when `i == 1`. Therefore, the output will be `5 15`.
Which method is used to sort an array in Java?
View Answer
Correct Answer: C
The `Arrays.sort()` method is used to sort arrays in Java.
What is the index of the last element in an array of size 5?
View Answer
Correct Answer: A
In Java, array indices start at 0. Therefore, the index of the last element in an array of size 5 is 4.
What happens if you try to assign a value of a different type to an array element?
View Answer
Correct Answer: B
If you try to assign a value of a different type to an array element, it results in a compilation error in Java.
How can you convert an array to a list in Java?
View Answer
Correct Answer: D
You can use the `Arrays.asList()` method to convert an array to a list in Java.
Which of the following is correct about arrays in Java?
View Answer
Correct Answer: D
Arrays in Java are passed by reference, meaning changes to the array inside a method will reflect outside the method as well.
What is the result of the following code?
int[] arr = {5, 10, 15};
System.out.println(arr[arr.length - 1]);
View Answer
Correct Answer: C
The expression `arr.length – 1` refers to the last index of the array, which is 2. Therefore, the value at index 2 is 15.
What will be the output of the following code?
int[] arr = new int[3];
arr[0] = 1;
arr[1] = 2;
arr[2] = 3;
System.out.println(arr[5]);
View Answer
Correct Answer: B
Array indices in Java are 0-based. The array has only 3 elements, so trying to access index 5 will throw an ArrayIndexOutOfBoundsException.
Which of the following is the correct way to create a multidimensional array with 2 rows and 3 columns in Java?
View Answer
Correct Answer: A
The correct way to create a 2D array with 2 rows and 3 columns is `int[][] arr = new int[2][3];`.
What is the value of arr[0] after executing the following code?
int[] arr = {1, 2, 3};
arr = new int[2];
View Answer
Correct Answer: B
After the second line of code, the array is reinitialized with size 2. The default value of integer array elements is 0, so `arr[0]` will be 0.
What happens if you try to access an uninitialized element of an array in Java?
View Answer
Correct Answer: B
In Java, uninitialized elements of an array are assigned default values, such as 0 for integers and null for object references.
What is the output of the following code?
int[] arr = {1, 2, 3, 4};
arr[0] = 5;
arr[1] = arr[0] + arr[3];
System.out.println(arr[1]);
View Answer
Correct Answer: D
The value of `arr[1]` becomes the sum of `arr[0]` (which is 5) and `arr[3]` (which is 4). Therefore, `arr[1]` becomes 9.
What is the result of the following code?
int[] arr = {1, 2, 3, 4};
arr = new int[5];
System.out.println(arr[4]);
View Answer
Correct Answer: C
When an array is initialized with a size of 5, all its elements are set to their default values (0 for integers). `arr[4]` will be 0.
Which of the following will cause a compile-time error?
View Answer
Correct Answer: C
Option 3 causes a compile-time error because the array must be initialized with curly braces `{}` directly after its declaration. `int arr[5] = {1, 2, 3};` is incorrect.
What is the output of the following code?
int[] arr = new int[3];
System.out.println(arr[2]);
View Answer
Correct Answer: C
Array elements are initialized to 0 by default, so `arr[2]` will output 0.
Which of the following is true about arrays in Java?
View Answer
Correct Answer: C
In Java, once an array is created, its size is fixed and cannot be changed. Option 2 is correct.
What will happen if you attempt to access a null array in Java?
View Answer
Correct Answer: C
If you try to access an array that is null, it will result in a `NullPointerException`.
What is the output of the following code?
int[] arr = {1, 2, 3, 4};
arr = new int[4];
System.out.println(arr[0]);
View Answer
Correct Answer: A
When a new array is created with the same size, all elements are initialized to their default value, which is 0 for integers.
How can you initialize a 2D array with the following values: `{{1, 2}, {3, 4}}`?
View Answer
Correct Answer: C
The correct way to initialize a 2D array with the values `{{1, 2}, {3, 4}}` is `int[][] arr = {{1, 2}, {3, 4}};`.
What is the result of the following code?
int[] arr = new int[3];
arr[0] = 1;
arr[1] = 2;
arr[2] = 3;
System.out.println(Arrays.toString(arr));
View Answer
Correct Answer: B
The `Arrays.toString()` method converts the array into a string representation. The output will be `[1, 2, 3]`.
What is the output of the following code?
int[] arr = {1, 2, 3};
arr = new int[]{4, 5, 6};
System.out.println(arr[0]);
View Answer
Correct Answer: C
The array is reinitialized with the values `{4, 5, 6}`, so `arr[0]` will output `4`.
Which of the following will correctly copy the elements of one array to another?
View Answer
Correct Answer: C
The correct method to copy an array is `Arrays.copyOf(arr2, arr2.length);`.
Which of the following will print the elements of a 2D array in a single line?
View Answer
Correct Answer: B
The `Arrays.deepToString()` method prints the elements of a 2D array in a readable format.
What will happen if you try to access an index outside the bounds of a 2D array?
View Answer
Correct Answer: D
Attempting to access an index outside the bounds of a 2D array will result in an `ArrayIndexOutOfBoundsException`.
What is the output of the following code?
int[][] arr = {{1, 2}, {3, 4}};
System.out.println(arr[1][1]);
View Answer
Correct Answer: A
The value at index `[1][1]` in the 2D array is `4`.
What is the result of calling `Arrays.sort()` on a non-sorted array?
View Answer
Correct Answer: B
The `Arrays.sort()` method sorts the elements of an array in ascending order.
What is the result of trying to assign a new value to an element of a final array reference?
View Answer
Correct Answer: B
Even though an array reference is `final`, you can still modify its elements. The `final` keyword only prevents reassigning the reference itself.
Leave a Reply